
To remove a file from Git and your local filesystem, run the git rm command and specify the file name.
git rm <file-name>
To remove a folder, use the -r option to recursively delete the subfolders and files of the directory:
git rm -r <folder-name>
You can also remove a file from Git but keep it locally by specifying the –cached option in the git rm command.
git rm --cached <file-name>
This is a comprehensive guide to deleting a file in Git. You will learn how to delete files from both your Git repo as well as the entire filesystem or even the entire commit history. You’ll also see examples of how to untrack files by removing them from Git but not from the project. The theory is backed with concrete examples with real Git projects to support understanding.
Let’s jump into it.
Delete Files with “git rm” Command
To delete a file from your Git repo, run the git rm command followed by the name of your file.
git rm <file-name>
Where you should replace the <file-name> with the name of the file or the path to the file you want to delete.
The “git rm” command removes the file from both the repository as well as the filesystem.
This command adds the deletion to the “staged” area of the project. To finalize the deletion of the file, you need to remember to commit the change.
Example
Let’s take a look at a concrete example with a real example repository to support understanding how files are deleted from Git.
In this example, I have a repository with three sample files, file1.txt, file2.txt, and file3.txt.

$ ls file1.txt file2.txt file3.txt README.md test.txt
Now let’s remove file1.txt from the repository and from the filesystem:
$ git rm file1.txt
Then, let’s check the status of the project using git status:

As you can see, file1.txt is now gone. Notice that the file still exists in the Git project index. If you’re sure you want to delete the file from Git as well, run git commit.
$ git commit -m "Remove file1.txt from the project"
Once you run this command, the file no longer exists in the Git repo or the file system.
By the way, there’s still a way to get the deleted file back by resetting to the previous commit with git reset –soft HEAD~1. This command undoes the last commit and puts the deletion back to staged. To unstage (i.e. to get the file back) run git restore –staged <file>.
Delete Folders “git rm -r”
Sometimes you might not only want to get rid of a file but entire directories of files. In this case, it’s not enough to run the git rm command as-is. This is because if you want to remove a directory, the removal must be recursive, that is, it must remove files and subfolders inside the directory.
To remove a folder from Git, run the git rm command with the -r option (for recursive deletion):
git rm -r <folder-name>
After running this command, the folder is removed from the filesystem, but it still lives in the Git repo. To remove it from the repo, you need to commit the change the same way you did earlier!
Example
As always, let’s take a look at an example of removing a folder from a Git repo.
Here’s a sample project with a subfolder called samples.

The samples folder has two files called file4.txt and file5.txt.
Let’s remove this folder from the Git project and the filesystem using git rm with the recursive option -r:
$ git rm -r samples
Here’s what you get when running this command and checking the status of the project:

As you can tell from the output, the command successfully deletes files file4 and file5 from the samples folder (along with the folder itself). The git status verifies this as it shows there are two files that are going to be removed.
Also, even though it’s not clear from the messages, the samples/ folder itself is removed too.

Remember, because you used git rm -r, the files aren’t yet removed from the Git project but the filesystem instead. To delete the folder from Git, remember to commit!
$ git commit -m "Remove the samples folder"
Now the folder and its contents are gone for good.
Delete from Git Repo Only
Sometimes you might want to delete a file from the Git project but not from the filesystem.
In this case, you need to run git rm with the –cached option.
git rm --cached <file>
Example: Stop Tracking a File
Let’s have a look at a concrete example to support understanding deleting files with the –cached option in Git.
Here’s a Git project that has a file called data.txt.
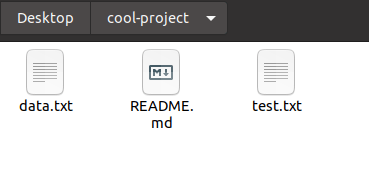
Previously, this file has been tracked, but now you want to stop tracking it but keep it locally in your filesystem. To do this, let’s run the git rm command with the –cached option.
$ git rm --cached data.txt
Now, let’s check the status of the project and the folder of the project:

The file is still there in the local folder, but deleting the file from Git is now staged. To delete the file from Git (and keep it locally) you still need to commit the changes.
$ git commit -m "Untrack the data.txt file"
After running this command, let’s check the status again.

Hmm… there the file still is! But this makes sense. You removed the file from being tracked by Git but as you wanted, the file still exists in the project. So it makes sense that Git shows it in the untracked files section.
To stop tracking the file (and to avoid accidentally adding it with git add), let’s create an invisible .gitignore file and add data.txt there.
I’m going to do this with Terminal to keep it short. You can do it any way you want as long as you end up with a file called .gitignore that lists the file you want to stop tracking.
$ touch .gitignore $ echo "data.txt" > .gitignore $ cat .gitignore
Now that you’ve placed the “data.txt” inside a file called .gitignore, Git knows to ignore it from the projects.
Let’s run git status again:

Now there’s no trace of the data.txt anymore as we added it to the .gitignore file.
Let’s commit the change and we’re no longer tracking the local data.txt file in the Git project:
$ git add . $ git commit -m "Add gitignore and stop tracking data.txt"
Speaking of making Git forget previously tracked files now in .gitignore, feel free to read this article to find a more elegant approach.
How to Delete Files from Git History
Sometimes you might want to delete a file from the entire Git history so that no-one can see such a file ever existed.
With what you’ve learned thus far, you can only remove files such that they are still accessible and visible in the commit history.
But to remove a file such that no one can see it even in the Git history, you need to run a slightly longer git filter-branch command:
git filter-branch --force --index-filter --prune-empty "git rm --cached --ignore-unmatch <file>" revision
This is a long command, so let’s break it down into smaller chunks:
- –force option forces the filter-branch to run even when it might not want to (e.g. because of temporary directories)
- –index-filter rewrites the Git index. This removes the file from the history.
- –prune-empty avoids leaving empty commits in the repository because of the removed files.
- “git rm –cached –ignore-unmatch <file>” is the command run on each matching branch, revision, and commit. In this case, it removes the file from the repo and ignores unmatching files.
- revision is the commit from which you want to start running the command (e.g. HEAD).
Example
Let’s see a concrete example of removing a file from the Git history.
In my example project, I have removed a file called file1.txt file from the repo. But by traversing commits back, this file is still accessible even though it’s gone.

Now I’m going to remove the file1.txt from the entire commit history by running:
$ git filter-branch --force --prune-empty --index-filter "git rm -r --cached --ignore-unmatch file1.txt" HEAD
After running this command, the commit history no longer has a file called file1.txt.

Removing a file from history like this might cause a mangled history rewrites. As you can see, the commits have different hashes at the moment, so the command basically rewrote the entire commit history of the project, even though the contents of the commits remain the same. To avoid trouble with these types of issues, you can use an alternative like “git filter-repo“.
Summary
Today, you learned how to delete files from Git.
More often than not, you should delete files with git rm <file> command after which you need to commit the deletion. This deletes the file both in the filesystem and from the Git project. To remove a folder, use the -r option for recursion git rm -r <folder-name>.
To remove a file from Git but not from the filesystem on your computer, do the git rm –cached <file> command. This removes the file from Git but leaves it as an untracked file in the system. To ignore the untracked file, add it to the .gitignore file and commit the change.
To remove a file from the entire project history in Git, use the rather lengthy git filter-branch command. Notice that this command rewrites history and might cause a mangled history rewrites.
Thanks for reading. Happy coding!