In JavaScript, the call()
and apply()
methods are both used to borrow and call a function on another object. The only difference between them is the way they accept arguments:
- The
call()
method takes the arguments as traditional function arguments separated by commas. - The
apply()
method takes the arguments as an array.
Both methods are useful when borrowing functionality. To call a function of one object on another object you can use call()
or apply()
.
This is a comprehensive guide to understanding what are the call()
and apply()
methods in JavaScript and how they differ from one another. Chances are you aren’t quite familiar with either method so let’s take a quick look at the call()
and apply()
methods separately before discussing the differences.
The call() Method on JavaScript
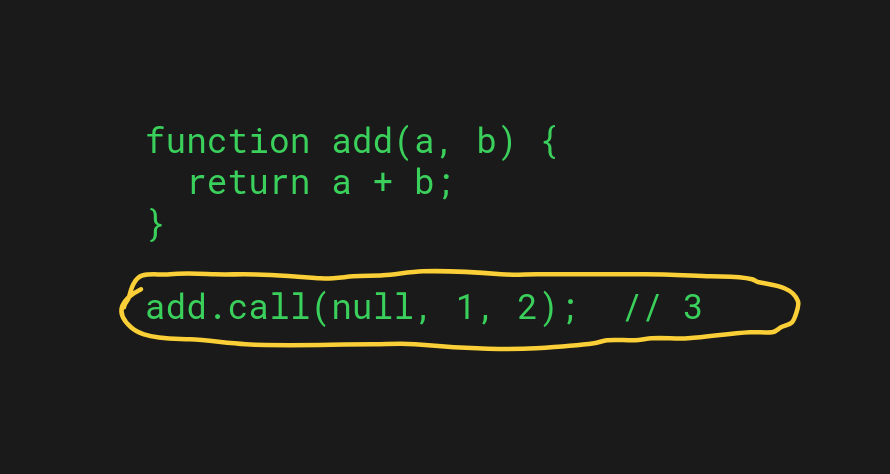
In JavaScript, the call()
method is used to call a function with a specified this
value and arguments provided individually. It is a method that is available on all functions in JavaScript, and it can be used to invoke the function and specify the value of this
inside the function.
Let’s see a couple of examples to support your understanding.
Example 1
For example, let’s call add()
function on two numbers.
function add(a, b) { return a + b; } console.log(add.call(null, 1, 2)); // 3
Output:
3
In the example above, the call()
method calls the add()
function and specifies null
as the value of this
into the add()
function.
The call()
method also takes arguments 1 and 2 as a comma-separated list. This produces the result of 3
, which is the sum of 1
and 2
– nothing too fancy, is it?
At this point, you may wonder why such a hassle to call a function. Why couldn’t you call the function directly instead of doing it via the call()
method?
The call()
method is often used in JavaScript when you want to borrow a method from one object and use it on another object.
Notice that the above example is essentially the same as directly calling the add()
function.
function add(a, b) { return a + b; } console.log(add(1, 2)); // 3
Let me show you another example that illustrates the function-borrowing aspect.
Example 2
For example, suppose you have two objects, obj1
and obj2
, and you want to use the add()
method from obj1
on obj2
.
You could do this using the call()
method, like this:
const obj1 = {name: "Alice"} const obj2 = {name: "Bob"} obj1.add = function(a, b) { console.log(this.name) return a + b; } // Using the call() method console.log(obj1.add.call(obj2, 1, 2)); // 3
Output:
Bob 3
In this example, the add()
method is borrowed from obj1
and used on obj2
. Here, the call()
method specifies the value of this
within the function add()
which in this case is the obj2
. Besides, we pass the arguments to the function to perform the addition operation on.
Notice how the output says “Bob
” even though initially the method belongs to obj1
whose name is “Alice
“. This is because the this
value is obj2
in the add()
method when using the call()
method the way we did above, if that makes sense.
The apply() Method on JavaScript

In JavaScript, the apply()
method is almost identical method to the call()
method. It’s used to call a function with a specified this
value and arguments provided as an array. The apply()
is a method that is available on all functions in JavaScript.
Let’s also see a couple of examples to better understand how this method works, although it’s very similar to the call()
method.
Example 1
For example, let’s add two numbers with add()
function via apply()
method:
function add(a, b) { return a + b; } console.log(add.apply(null, [1, 2])); // 3
Output:
3
In the example above, the apply()
method invokes the add()
function (and for the sake of simplicity, it specifies null
as the value of this
within the function).
The apply()
method takes the arguments as an array. This produces the result of 3
, which is the sum of 1
and 2
that are given in the array.
Similar to the call()
method, the apply()
method is useful when you want to borrow a method from one object to another.
Example 2
For example, suppose you have two objects, obj1
and obj2
, and you want to use the add()
method from obj1
on obj2
.
You could do this using the apply()
method, like this:
const obj1 = {name: "Alice"} const obj2 = {name: "Bob"} obj1.add = function(a, b) { console.log(this.name) return a + b; } // Using the call() method console.log(obj1.add.apply(obj2, [1, 2])); // 3
Output:
Bob 3
In this example, the add()
method is borrowed from obj1
and used on obj2
. The apply()
method sets the this
value for the function. In this case, this
refers to obj2
inside the add()
function. So far it’s exactly how the call()
method works. The only difference being in that the arguments are given as an array.
Using the apply()
method in this case works but is not the right choice because you already know the function add()
expects two arguments that it adds together. If you didn’t know the number of arguments in advance, then passing them as an array would make more sense, that is, using the apply()
method.
call() vs apply()

In the previous sections, you learned what the call()
and apply()
methods are and how they work.
The key difference between call()
and apply()
is very subtle.
The call()
method takes arguments as traditional function arguments separated by commas. The apply()
method takes the function arguments as an array of an arbitrary number of arguments.
Here’s an example that illustrates the small difference between the methods:
function add(a, b) { return a + b; } // Using the call() method console.log(add.call(null, 1, 2)); // 3 // Using the apply() method console.log(add.apply(null, [1, 2])); // 3
Output:
3 3
All in all, the call()
and apply()
methods are used to borrow a method from one object to another.
The call()
method is used when you know the exact number and types of arguments that the function expects, while the apply()
method is useful when you don’t know the exact number or types of arguments that the function expects, or when you want to pass the arguments as an array.
Summary
Overall, the main difference between the call()
and apply()
methods is the way they pass arguments to the function.
The call()
method takes the arguments as a comma-separated list, while the apply()
method takes the arguments as an array. Both methods are useful in different situations, and which one you should use will depend on the specific needs of your code.
Thanks for reading. Happy coding!