When dealing with strings in JavaScript, it’s common for you to need to replace specific characters or substrings with other values.
This guide shows you three ways to replace all string occurrences in JavaScript. Also, you learn why some approaches can be better than others.
Here’s a quick look at the three approaches you are going to learn:
const message = "this-is-just-a-test" // 1: The split-and-join approach message.split("-").join(" ") // 2: The replace() method with global regexp message.replace(/\-/g, " ") // 3: The replaceAll() method message.replaceAll("-", " ")
For instance, the replaceAll() method is inarguably the best way to replace all occurrences. But it’s not yet supported everywhere, so it can cause some hiccups.
Anyway, let’s jump into the solutions.
1. The Split-and-Join Approach
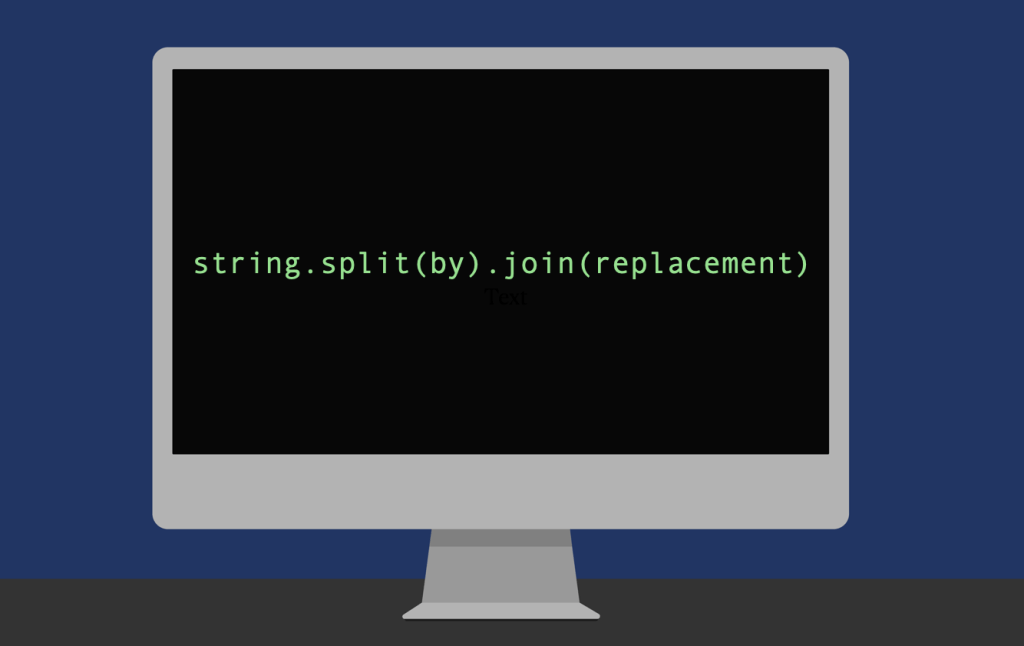
One popular approach to replacing all occurrences of a string inside a string is by using the split-join approach.
The idea of the split-join approach is to:
- Split the string into an array of strings based on a separator value, such as a blank space. This happens with the split() method.
- Join the array of strings back to one long string by using a specific separator value, such as a dash. This happens with the join() method.
Here’s an example.
Let’s replace each dash with a blank space in the example string:
const message = "this-is-just-a-test" const nodashes = message.split("-").join(" ") console.log(nodashes)
Output:
this is just a test
Now, let’s take a quick look at how the code works.
The message.split(“-“) part splits the string into an array of strings using the dashes as the “splitting points”. The method leaves out the dashes from the result array. This means the result is an array [“this”, “is”, “just”, “a”, “test”].
Then you call .join(” “) on this result array. This loops through the array of strings and joins the strings by using a blank space ” “ as a separator. This gives you a string “This is just a test“. Now you’ve successfully replaced all dashes with blank spaces.
If you’re looking for a solution that works, then there you have it!
Notice that this is not the most optimal way to do things because you first turn the string into an array and then back to a string.
That’s why I’ll show you two more approaches.
2. The replace() Method
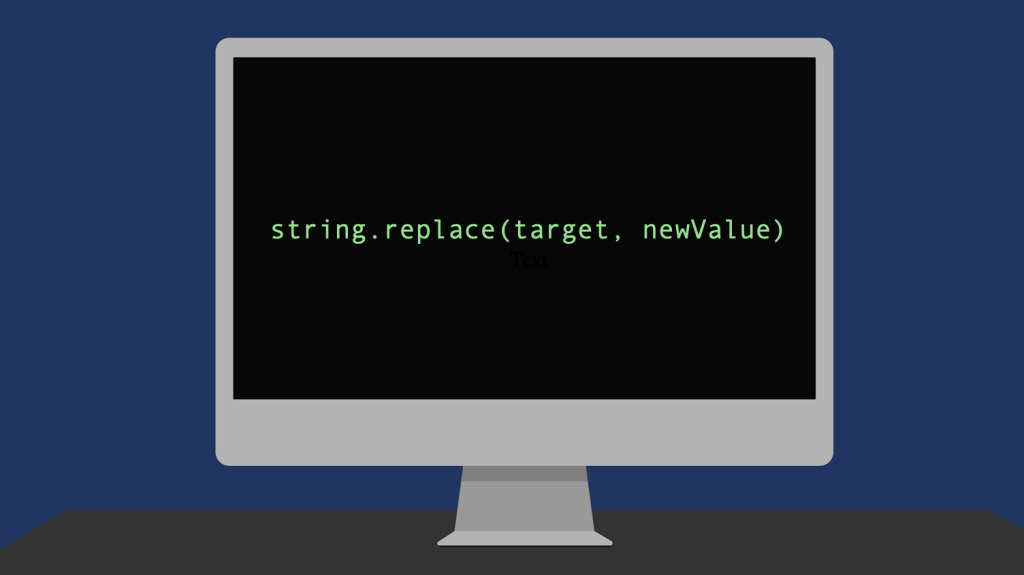
Another way to replace occurrences of a string in JavaScript is by using the replace() method.
But here’s the problem: The replace method only replaces the first occurrence of a string inside a string!
For example, let’s try to replace all the dashes with a blank space:
const message = "this-is-just-a-test" const nodashes = message.replace("-", " ") console.log(nodashes)
Output:
this is-just-a-test
Not great!
To replace all the occurrences, use a global regular expression as the first argument of the replace() method.
Assuming you understand the basics of regular expressions, here’s an example code that replaces all the dashes from a string with blank spaces:
const message = "this-is-just-a-test" const nodashes = message.replace(/\-/g, " ") console.log(nodashes)
Output:
this is just a test
The above regular expression /\-/g is an example of a global regexp. The global means it matches all occurrences. The \- tells the regular expression that it’s looking to match with dashes.
This is a more consistent way to replace string occurrences than the first approach. But this requires regexp knowledge, which can take a while to learn. That’s why there’s still one approach you might be interested in.
3. The replaceAll() Method

Finally, the best solution for replacing all occurrences of a string is by using the new replaceAll() method. This is a method that’s consistent, intuitive, and easy to use.
The replaceAll() method takes the string to be replaced as the first argument and the replacement string as a second argument.
The reason why this approach is the last one on the list is because of the limited browser support.
Anyway, here’s an example code that replaces all the dashes of a string with blank spaces using the replaceAll() method:
const message = "this-is-just-a-test" const nodashes = message.replaceAll("-", " ") console.log(nodashes)
Output:
this is just a test
Mind the limitations in the use of the replaceAll() method.
Conclusion
Today you learned how to replace all occurrences in a string in JavaScript.
To take home, there are three options:
- The split-join approach. The idea is to first split the string into an array of strings using a separator. Then join the result array back to a string with a replacement value.
- The replace() method with global regular expressions. This approach uses regular expressions to match each occurrence and replace them with another string.
- The new replaceAll() method (limited support). This is the best and simplest approach to replacing all occurrences in a string. Specify the first argument as the occurrence you want to replace and the second argument as the replacement string.
Thanks for reading. Happy coding!