
The Short Answer
There are several ways to check if a JavaScript string contains a substring. Here’s a short summary of the most notable ones.
1. string.indexOf()
Use the indexOf()
method. This method returns the index of the first occurrence of the substring in the string, or -1 if the substring is not found.
For example, let’s check if “word” exists in “Hello world!”:
var str = "Hello world!"; if (str.indexOf("world") >= 0) { console.log("There's a substring") }
Output:
There's a substring
2. string.includes()
Use the includes()
method. This method returns a boolean value indicating whether the string contains the substring or not.
For example, let’s check if “word” exists in “Hello world!”:
var str = "Hello world!"; if (str.includes("world")) { console.log("There's a substring") }
Output:
There's a substring
3. string.search()
Using the search()
method: This method is similar to indexOf()
, but it supports regular expressions, allowing for more advanced substring matching.
For example, let’s check if “word” exists in “Hello world!”:
var str = "Hello world!"; if (str.search(/world/) >= 0) { console.log("There's a substring") }
Output:
There's a substring
4. string.match()
Using the match()
method: This method searches the string for a match against a regular expression, and returns an array containing the matched substrings, or null
if no match is found.
For example, let’s check if “word” exists in “Hello world!”:
var str = "Hello world!"; if (str.match(/world/)) { console.log("There's a substring") }
Output:
There's a substring
This is a comprehensive guide to checking if a string has a substring in JavaScript.
In case you found what you were looking for in the above answers, feel free to stop reading right here. But this is a wonderful chance to learn JavaScript at the same. To learn more about the above useful string methods and how they work, make sure to read along.
Let’s jump into it!
1. The string.indexOf() Method

The indexOf()
method in JavaScript is used to search for a specified string in a given string and return the index at which the specified string is found. If the specified string is not found, the indexOf()
method will return -1.
For example:
var str = "Hello, world!"; var n = str.indexOf("world"); var m = str.indexOf("test"); console.log(n); // Output: 7 console.log(m); // Output: -1
The indexOf()
method can also take an optional second argument, which specifies the index at which the search should begin.
For example:
var str = "Hello, world!"; var n = str.indexOf("world", 5); console.log(n); // Output: 7
In the example above, the search for the string “world” begins at index 5 of the string “Hello, world!”. Since “world” appears at index 7 (you can count it with your fingers to see that’s indeed the case), that is what the indexOf()
method returns.
2. The string.includes() Method

The includes()
method in JavaScript checks if a string contains a specified substring. It returns true
if the specified substring is found in the given string, and false
if it is not.
For example:
var str = "Hello, world!"; var isIncluded = str.includes("world"); console.log(isIncluded); // Output: true
In the example above, the includes()
method checks if the string “Hello, world!” contains the substring “world”. Since it does, the method returns true
.
The includes()
method can also take an optional second argument, which specifies the index at which the search should begin.
For example:
var str = "Hello, world!"; var isIncluded = str.includes("world", 5); console.log(isIncluded); // Output: true
Here the substring world is sought after the 5th index (6th character) of the string. Since “world” occurs at the 7th index it means there’s a substring “world” after starting the search from index 5.
Note that the indexOf()
method can be used to achieve the same result as the includes()
method. But the indexOf()
returns the index at which the specified substring is found, whereas includes()
returns a boolean value. So, it depends on the requirement of which method to use.
3. The string.search() Method
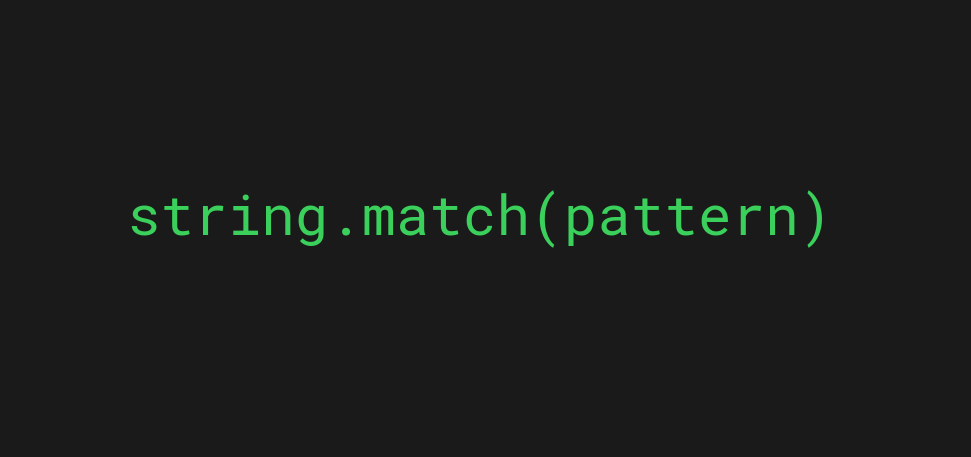
The third option to search for substrings in JavaScript is by using the search()
method. This method tries to find a specified substring in a string. It returns the index at which the specified string is found. If the specified string is not found, the search()
method will return -1.
For example:
var str = "Hello, world!"; var n = str.search("world"); console.log(n); // Output: 7
The search()
method can also accept a regular expression as a parameter. In this case, the method will return the index at which the specified regular expression pattern is found in the given string.
For example:
var str = "Hello, world!"; var n = str.search(/[aeiou]/); console.log(n); // Output: 1
In the example above, the search()
method searches the first lowercase vowel (“a”, “e”, “i”, “o”, “u”) in the string “Hello, world!”. It returns the index at which the first match is found, which in this case is 1 (the “e” in “Hello”).
As you can see, the search()
method not only achieves the same result as the indexOf()
method, but also understands regular expressions. As such, the search()
method is more powerful and flexible than the indexOf()
method.
4. The string.match() Method

Last but not least, let’s have a look at a similar method with search()
called match()
. The match()
method searches for a substring in a string and returns an array of matching substrings. If no matches are found, the match()
method will return null
. This method also accepts regex inputs.
Here is an example of how you can use the match()
method:
var str = "Hello, world!"; var matches = str.match("world"); console.log(matches); // Output: ["world"]
Here the match()
method searches for the string “world” in the string “Hello, world!”. The method returns an array containing the matching string, which in this case is ["world"]
.
The match()
method can also accept a regular expression as a parameter. In this case, it returns an array the substrings in the given string that match the specified regular expression pattern.
For example, let’s search for all the lowercase vowels in a string:
var str = "Hello, world!"; var matches = str.match(/[aeiou]/g); console.log(matches); // Output: ["e", "o", "o", "a"]
Here, the match()
method searches for a pattern that matches any lowercase vowel in the string “Hello, world!”. The method returns an array of all the matching substrings. In this case, the result is ["e", "o", "o", "a"]
.
The match()
method can be used to achieve the same result as the search()
method, but it returns an array of matching substrings instead of the index at which the first match is found. Sometimes this makes the match()
method more useful for extracting information from a string.
string.search() vs string.match()
The key difference between the match()
and search()
methods in JavaScript is how they return the results of the search.
The search()
method returns the index at which the first match is found, whereas the match()
method returns an array of all the matching substrings.
Although you already saw a couple of examples, here’s one more that demonstrates the difference between the two:
var str = "Hello, world!"; var n = str.search("world"); console.log(n); // Output: 7 var matches = str.match("world"); console.log(matches); // Output: ["world"]
Thanks for reading. Happy coding!