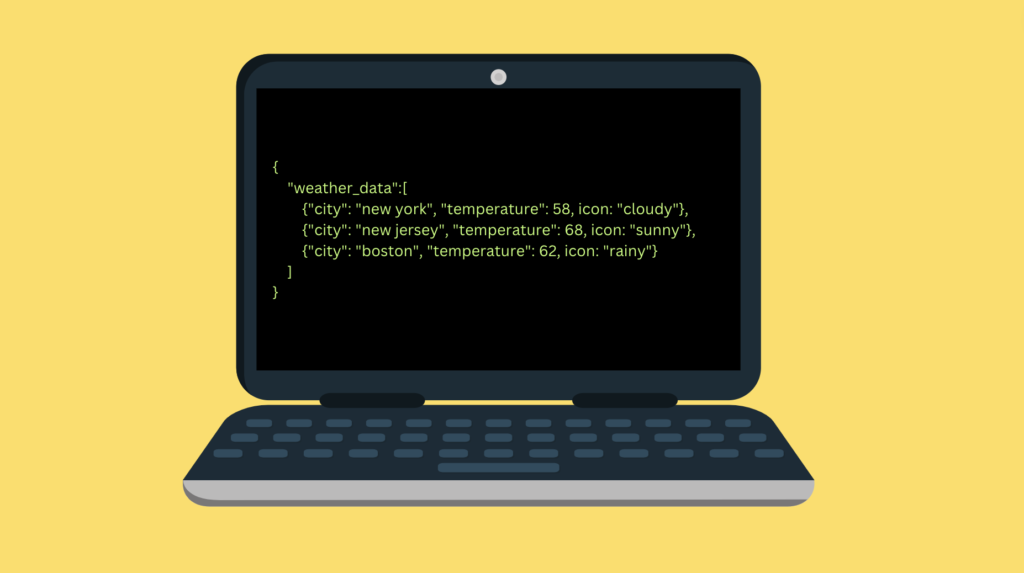
JSON is a text-based lightweight data format for transmitting data over the internet.
JSON is supported by most modern programming languages. This makes it a highly usable data format on almost any coding project.
A typical use case for JSON data is sending data over the internet as requests and responses.
For example, a weather app receives the local weather data as JSON before showing it on your smartphone’s screen.
This is a comprehensive guide to JSON.
You will learn about JSON rules, origin, and alternatives. Besides, you will see a slightly more technical example where I create an HTML table from JSON data.
As a developer, learning JSON is important because it’s almost impossible to avoid working with JSON at least at some point in your career.
What Is JSON?
JSON stands for JavaScript Object Notation. It’s a commonly used data format in data transmission.
Exchanging data between a server and a client is a common task in applications and programs.
For example, a weather app can send your location data to a weather server that then sends back the local weather data to you.
This is how a typical weather app works behind the scenes.
Notice that every single byte of data transmitted over the internet requires bandwidth.
Naturally, the transmitted data packets should always take as little space as possible. Thus, the data sent should be formatted in an optimal way.
This is where JSON is used. JSON is a lightweight text-based data format used in data transmission.
JSON data is readable to developers and takes little space when sent over the internet. This makes it a perfect data format for many applications and projects.
Notice that for a long time, XML was the de-facto data format for transmissions. But during the early 2000s, JSON took over as a great alternative to XML!
Here’s an example of how JSON data might look like:
{ "weather_data":[ {"city": "new york", "temperature": 58, icon: "cloudy"}, {"city": "new jersey", "temperature": 68, icon: "sunny"}, {"city": "boston", "temperature": 62, icon: "rainy"} ] }
Take a look at the JSON data above. I bet you get a pretty good idea of what the data is all about even if you have never seen JSON before. This demonstrates how readable the JSON data is and how it makes developers’ life easier because it’s easy to comprehend.
Let’s take a closer look at the JSON data contents and the rules that govern JSON formatting.
JSON Is All About Key-Value Pairs
JSON or JavaScript Object Notation is a text-based data format. It’s a collection of key-value pairs. In JSON:
- Each key should be a String
- Each value can be any of the following data types:
- Number. For example, 432
- String. For example, “Alice“
- Boolean. Either true or false
- Array (or list). For example, [“A”, “B”, “C”]
- null. An empty value.
Important JSON Rules
Here are the most important rules of JSON formatting.
- JSON data can have two main formats:
- Key-value pairs governed by curly braces {…}
- A list of previously mentioned key-value pairs, separated by a comma and enclosed by square brackets [{…}, {…}, {…}]
- In JSON, the keys must be wrapped inside double quotation marks. For example, “weather”.
- Key-value pairs should be separated by colons. For example, “weather”: “cold”.
- Multiple key-value pairs must be separated by commas. For example, {“weather”: “cold”, “precipitation”: 1.4}
- JSON doesn’t support “code comments”.
JSON is typically formatted to stretch across multiple lines. Each JSON object is indented nicely to make the data more readable. But this is not mandatory. When sending JSON data over the internet, the formatting is typically discarded to save bandwidth.
Let’s see some concrete examples of JSON data to support understanding the rules and formatting.
Example JSON Data
Remember, JSON is just a structured text file with data in it. It’s almost like a text file but one that follows rather strict formatting guidelines.
Here’s some sample JSON that represents the data of some residents in the US. This piece of JSON illustrates the key rules of JSON:
[ { "name": "Alice", "age": 20, "city": "New York", "hobbies": ["Jogging", "Gym"], "married": false }, { "name": "Bob", "age": 40, "city": "Boston", "hobbies": ["Gym", "Disc Golf"], "married": true }, { "name": "Charlie", "age": 29, "city": "Los Angeles", "hobbies": ["Soccer", "Jogging", "Fishing"], "married": true } ]
Let’s take a closer look at this data and some of its nuances.
The entire JSON data in the above example represents the data of three individuals. The above JSON object is a list that contains three inner JSON objects. So the structure of the JSON data is [{…}, {…}, {…}] but because there’s lots of data, it’s broken down into multiple lines.
Now let’s inspect the JSON data of the first person, that is Alice:
- “name”: “Alice”. The value “Alice” is wrapped around double quotes because it’s a string.
- “age”: 20. The value 20 is not wrapped around double quotes because it’s a number.
- “city”: “New York”. The value “New York” is in double quotes because it’s a string.
- “hobbies”: [“Jogging”, “Gym”]. Because there are many hobbies, you specify a list in which you specify the names of the hobbies as strings.
- “married”: true. In this case, the value is either true or false, so it’s a boolean and doesn’t need double quotes.
Notice how each key-value pair is followed by a comma. You must do this to include multiple key-value pairs in the JSON data.
JSON Usually Lacks Structure
JSON is a lightweight data format. Every character takes a bit of bandwidth when sent over the network. Even white spaces and line breaks require space.
When sending the data over the internet, redundant blank spaces and line breaks are bad because they eat up the bandwidth and slow down the requests. This is why usually JSON is sent as a messy blob of data that looks something like this:
[{"name":"Alice","age":20,"city":"New York","hobbies":["Jogging","Gym"],"married":false},{"name":"Bob","age":40,"city":"Boston","hobbies":["Gym","Disc Golf"],"married":true},{"name":"Charlie","age":29,"city":"Los Angeles","hobbies":["Soccer","Jogging","Fishing"],"married":true}]
This type of JSON is exactly the same JSON you saw earlier, but the line breaks and blank spaces are taken away from it.
This makes the JSON even lighter but also more unreadable.
If you have a long piece of JSON data, you might benefit from using a tool like JSON formatter (which is free).
For example, here’s a piece of unformatted input JSON data:

With the JSON formatter, you can make it structured and easy to navigate. Here’s what the formatted JSON looks like:
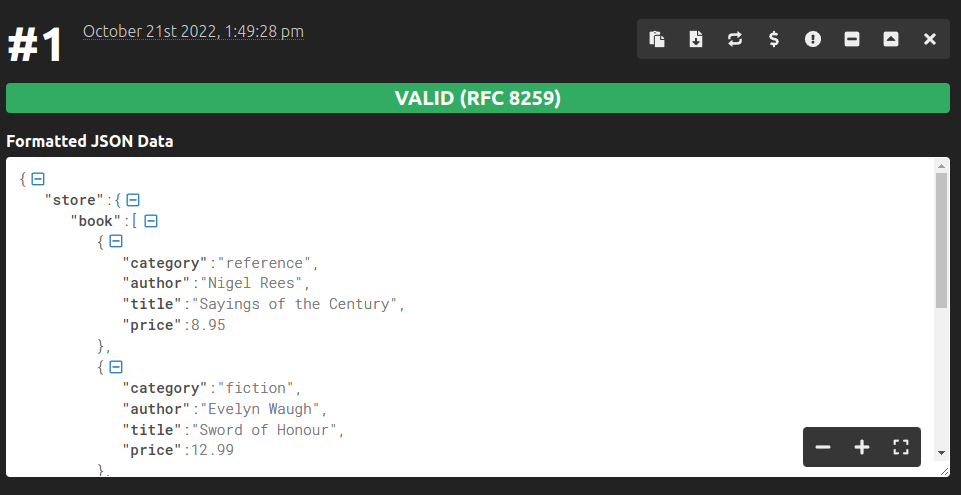
JSON’s Relation to JavaScript
JSON stands for JavaScript Object Notation and is indeed derived from JavaScript. As a matter of fact, every JSON object is also valid JavaScript code.
Even though JSON has relations to JavaScript, JSON is independent of any programming language!
Most modern programming languages support handling JSON data because JSON is such an optimal data format for data transmission. This is why you can use JSON with languages like Python, Swift, C++, and such.
Speaking of JavaScript, let’s take a look at an example with JSON and JavaScript.
JSON Example with JavaScript & HTML
If you’re completely new to JSON and data transmission, let me show you an example of how you could use JSON in an HTML page with JavaScript.
Assume you made an HTTP request to a web server and it returns the data of people as JSON. Instead of showing the data in a JSON format, you want to create a nicely formatted HTML table for your users.
Here’s the HTML table:
<div> <table id="peopletable" style="width:100%"> <tr> <th>Name</th> <th>Age</th> <th>City</th> <th>Hobbies</th> <th>Married</th> </tr> </table> </div>
And here’s the JavaScript file with the JSON response:
const response = [ { "name": "Alice", "age": 20, "city": "New York", "hobbies": ["Jogging", "Gym"], "married": false }, { "name": "Bob", "age": 40, "city": "Boston", "hobbies": ["Gym", "Disc Golf"], "married": true }, { "name": "Charlie", "age": 29, "city": "Los Angeles", "hobbies": ["Soccer", "Jogging", "Fishing"], "married": true } ] function addTable() { var table = document.getElementById("peopletable"); var tableBody = document.createElement('TBODY'); table.appendChild(tableBody); for (var i = 0; i < response.length; i++) { var tr = document.createElement('TR'); tableBody.appendChild(tr); for (const [key, value] of Object.entries(response[i])) { var td = document.createElement('TD'); td.width = '75'; td.appendChild(document.createTextNode(value)); tr.appendChild(td); } } } addTable();
The result of running this JavaScript code renders the JSON data in a nice table like this:

JSON Alternatives
JSON hasn’t always been the go-to data format for data transfer. Before the early 2000s, XML was the thing.
The key difference between XML and JSON is that:
- XML is a markup language.
- JSON is only a data format.
XML is less readable and takes more space than JSON. But XML is a markup language, you can do computations and processing with it. This is something you cannot do with JSON, which is merely a data format that contains the text.
So it’s really not fair to say JSON is a replacement for XML. In many cases, it is, but in some situations, you still need XML, for example, when you need to run code or processes among the data.
Here’s what XML looks like:
<weather> <location> <name>New York</> <temperature>58</temperature> <icon>cloudy</icon> <location/> <name>New Jersey</> <temperature>68</temperature> <icon>sunny</icon> <location> <name>Boston</> <temperature>62</temperature> <icon>rainy</icon> <location/> </weather>
Here’s a table that describes the key differences between XML and JSON.
JSON | XML |
---|---|
Easy to read thanks to key-value pairs and simple text format | Less easy to read because data is enclosed in markup tags. |
Less space is needed to represent data | More space is needed to represent data |
Quicker to parse thanks to text-based key-value pairs | Slower to parse because data needs to be extracted from tags |
Easy to create thanks to the easy syntax | Slightly more difficult to create thanks to the markup tags and a bit trickier syntax |
Supports a limited range of data types and doesn’t run code | More flexibility thanks to the markup language capabilities and the ability to run code |
Based on the JavaScript programming language | Based on SGML (Standard Generalized Markup Language) |
Doesn’t support comments | Supports comments |
Supports UTF-8 encoding only | Supports multiple encodings, such as UTF-16 |
Conclusion
Today you learned about JSONāthe most popular data format for transmitting data.
To take home, JSON is a text-based data format. JSON is lightweight which makes it great for transmitting data over networks. Moreover, JSON data is human-readable which makes it easy for developers to use.
Here’s an example of JSON data:
{ "weather_data":[ {"city": "new york", "temperature": 58, icon: "cloudy"}, {"city": "new jersey", "temperature": 68, icon: "sunny"}, {"city": "boston", "temperature": 62, icon: "rainy"} ] }
Practically all modern programming languages support JSON. All software developers need to learn how to use JSON as it’s practically impossible to avoid it.
The most notable use case for JSON is sending and receiving data over the internet.
For instance, a weather app on your smartphone receives the weather data as JSON over the internet and turns it into a nicely formatted weather UI.