
To get the length of a string in Swift, call the String.count property.
For example:
let myStr = "This is a test" let length = myStr.count print(length)
Output:
14
Replace ‘count’ with ‘length’ in Swift
Swift developers decided to call the length of a string ‘count’. However, if you prefer ‘length’ over ‘count’ you can change it by writing an extension to the String class.
Naively, you could write a method that accesses the count of the string:
extension String { func length() -> Int { return self.count } }
But this would mean you have to call the length with a set of parenthesis, unlike count.
let myStr = "Hello, world" print(myStr.length())
If you would like to call String.length without parenthesis similar to String.count, you can write an extension that utilizes computed properties.
Here is how to do it:
extension String { var length: Int { return self.count } }
Now it is possible to call String.length on any Swift string:
let myStr = "Hello, world" print(myStr.length)
Output:
12
Timeline of Swift String Length
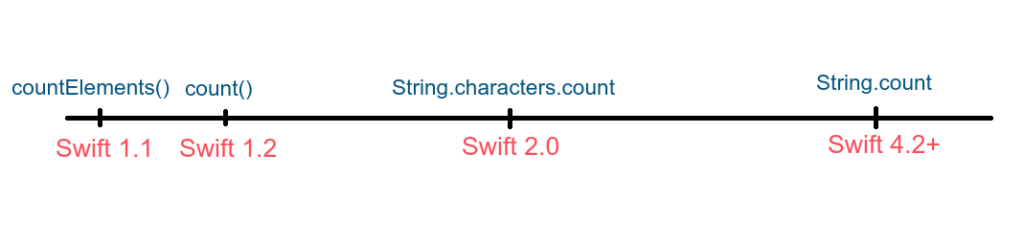
Swift 1.1
In Swift 1.1, counting characters of a string was possible using the countElements() function. This function returned the number of elements in a collection, such as a string:
let myStr = "Hello, world" let length = countElements(myStr) print(length)
Output:
12
Swift 1.2
In Swift 1.2 you could count the number of letters in a string with a count() function:
let myStr = "Hello, world" let length = count(myStr) print(length)
Output:
12
Swift 2.0
In Swift 2.0, the count() function was removed and was replaced by a member function String.characters.count.
For example:
let myStr = "Hello, world" let length = myStr.characters.count print(length)
Output:
12
Swift 4.2+
As of Swift 4.2, to get the length of a string, access the String.count member.
For example:
let myStr = "Hello, world" let length = myStr.count print(length)
Output:
12
Tip of the Day – String.isEmpty
If you need to find out if a string has exactly 0 characters, you could check String.count is 0:
let myStr = "" if myStr.count == 0 { // Do something }
But you can do this more conveniently by accessing the isEmpty property:
let myStr = "" if myStr.isEmpty { // Do something }