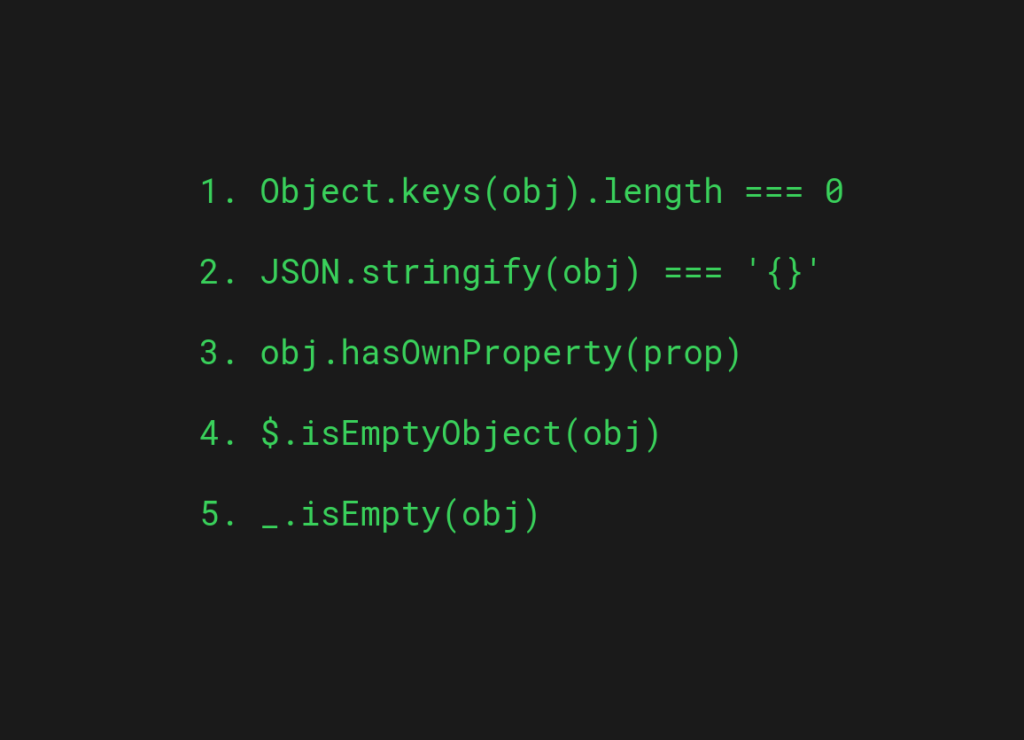
The easiest way to check if a JavaScript object is empty is by using the Object.keys
to check the length of properties to see if it’s 0.
function isEmpty(obj) { return Object.keys(obj).length === 0; }
This guide teaches you 5 ways to check if a JavaScript object is empty.
Although it only takes one, this is a great chance to learn more about JavaScript. Besides, if you’re using jQuery or lodash libraries, you might find it useful to learn you can use those to check if an object is empty.
5 Ways to Check If an Object Is Empty
Here’s a quick overview of the methods you can use to check if an object is empty in JavaScript:
- Object.keys
- JSON.stringify
- Loop object properties
- jQuery isEmptyObject()
- Lodash isEmpty()
Where the 4th and 5th approaches only really make sense if you’re using those libraries in your code already.
Let’s take a closer look at these approaches.
1. Object.keys
In JavaScript, the Object.keys
method returns an array of the object’s enumerable properties. The properties considered when using Object.keys
are those that can be accessed in a for-in loop, and those that have been assigned a non-symbolic, non-undefined value.
To use the Object.keys
method, you pass in the object that you want to get the properties of as the argument. The method then returns an array of strings, where each string is the name of one of the object’s enumerable properties.
To check if an object is empty in JavaScript using Object.keys
, you can use the following code:
function isEmpty(obj) { return Object.keys(obj).length === 0; }
To use this function, you can pass in any object that you want to check if it is empty.
For example:
function isEmpty(obj) { return Object.keys(obj).length === 0; } const obj = {}; const empty = isEmpty(obj); // true console.log(empty)
2. JSON.stringify
In JavaScript, JSON.stringify()
is a method that converts a JavaScript object or value to a JSON string. This method is often used when sending data to a server or when saving data to a file.
To check if a JavaScript object is empty, use the JSON.stringify()
method to convert a JavaScript object to a JSON string and check if the result is an empty JSON object ‘{}’.
For example:
const obj = {} // Convert the object to a JSON string const json = JSON.stringify(obj); // Check if the JSON string is empty if (json === '{}') { console.log('The object is empty'); }
3. Loop Object Properties
To test if a JavaScript object is empty, you can use a for-in loop to loop through the object’s properties. If the object has no properties, then it is empty.
Here is an example:
function isEmpty(obj) { for (var prop in obj) { if (obj.hasOwnProperty(prop)) { return false; } } return true; }
This function takes an object as its argument and returns true
if the object is empty and false
if it is not. It simply loops through the properties of the object and checks if they exist in the object. If none exists, the object is naturally empty.
4. jQuery (Library)
jQuery is a popular JavaScript library that makes it easier to add interactivity and other dynamic features to websites.
It allows developers to easily manipulate the HTML elements on a page and create animations, handle user events, and perform other common JavaScript tasks. jQuery is widely used and is considered one of the most important tools for front-end web development.
jQuery has a variety of powerful built-in functions you can use to save time when writing code. For example, you can create fade animations or similar using jQuery.
One built-in function that jQuery comes with is the isEmptyObject()
function. You can use this function to check if a JavaScript object is empty.
Notice that using this method does not make sense unless you’re using jQuery in your project already!
Here’s an example:
// create an object with some properties var obj = { name: 'John Doe', age: 30 }; // check if the object has any properties if ($.isEmptyObject(obj)) { console.log("Empty!") } else { console.log("Not empty") }
5. Lodash (Library)
Lodash is a JavaScript library that provides utility functions for common programming tasks.
It is a popular choice for front-end developers because it helps to reduce the amount of code to write, and makes it easier to work with arrays, objects, and other data types. With lodash, you can manipulate and iterate over arrays, objects, and strings, as well as functions for creating composite functions and working with asynchronous code.
To check if an object is empty using lodash, you can use the _.isEmpty()
method. This method returns true
if the object is empty, and false
otherwise.
Assuming you have lodash included in your project, here’s an example:
const obj = {}; console.log(_.isEmpty(obj)); // logs "true"
Thanks for reading. Happy coding!