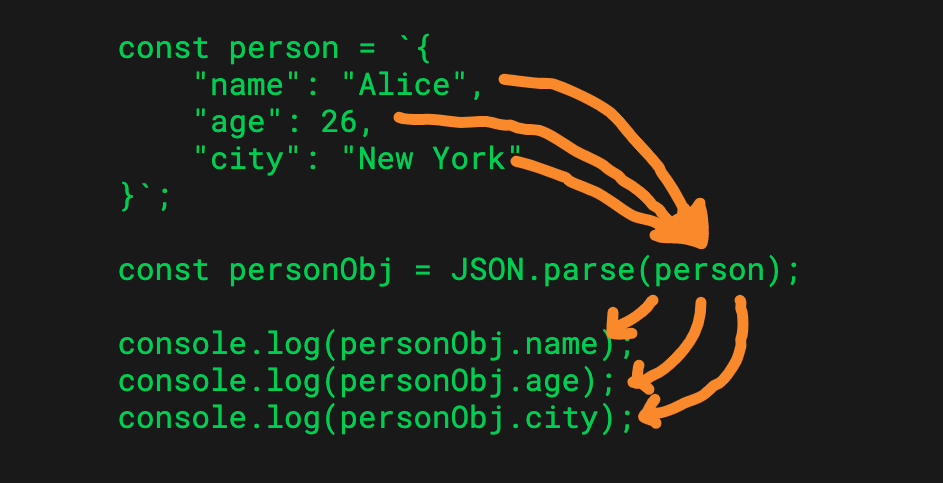
JSON is a popular text-based data format that is used to transmit data in network requests.
JSON has an easy-to-read syntax and it’s a lightweight data format. This is what makes JSON such a favorable data format.
As a web developer, dealing with JSON is almost impossible to avoid. When dealing with JSON, one of the most common tasks you need to do is parse the JSON data and handle potential errors.
This is a comprehensive guide to JSON parsing in JavaScript.
If you’re looking for a quick answer, use the JSON.parse() function.
For example:
const person = `{ "name": "Alice", "age": 26, "city": "New York" }`; const personObj = JSON.parse(person); console.log(personObj.name); console.log(personObj.age); console.log(personObj.city);
Output:
Alice 26 New York
JSON.parse() — How to Parse JSON in JavaScript
JavaScript has a built-in method JSON.parse() for parsing JSON strings. This method constructs a JavaScript object from a JSON string input.
As a matter of fact, based on the input JSON, the JSON.parse() function can return an Object, Array, string, number, boolean, or null.
There are two ways to call the JSON.parse() method:
- JSON.parse(text)
- JSON.parse(text, reviver)
The first approach is more common as you simply convert a JSON string to a JavaScript object. The second approach involves an optional reviver function.
Let’s take a closer look at the two main ways to call the JSON.parse() function.
1. JSON.parse(text)
The simplest and the most common way to call the JSON.parse() function in JavaScript is by passing it a JSON string argument.
For example, let’s parse a simple JSON string that represents a pet:
const pet = '{"name": "Luna", "age": 10}' const petObj = JSON.parse(pet); console.log(petObj.name); console.log(petObj.age);
Output:
Luna 10
The result of calling the JSON.parse() function this way returns a JavaScript object via which you can access the JSON key-value pairs with the dot notation.
Here are more examples that illustrate what different types of text inputs cause the JSON.parse() function to return.
JSON.parse("{}") // {} JSON.parse("false") // false JSON.parse('"Hello"') // "Hello" JSON.parse('[20,"Test",false]') // [ 20, 'Test', false ] JSON.parse("null") // null
2. JSON.parse(text, reviver)
Another way to call the JSON.parse() function is by passing it a second optional argument called reviver The idea of the reviver function is to perform an operation for the key-value pairs in the JSON data.
If you specify a reviver function, you must call it with the arguments
- key. The key is related to the value processed by the reviver.
- value. The value associated with the processed key.
Here’s the syntax for calling the JSON.parse() function with a reviver function:
JSON.parse(text, (key, value) => { /* statements */ })
To make any sense of these, you will need to see some examples.
For example, let’s convert the value associated with a key “age” to days. To do this, you need to specify a reviver function. The reviver multiplies the value by 365.25 if its key is “age” and the value is a number.
Here’s what the code looks like:
const pet = '{"name": "Luna", "age": 10}' const petObj = JSON.parse(pet, (key, value) => { if (key === "age" && typeof value === "number") { return value * 365.25 } else { return value } }); console.log(petObj.name); console.log(petObj.age);
Output:
Luna 3652.5
Now the age of the pet is expressed in days rather than years. (Notice how the name property remains untouched thanks to the if-else statement in the reviver function).
Limitations with JSON.parse() Function
There are two main limitations with the JSON.parse() function:
- JSON.parse() doesn’t accept trailing commas.
- JSON.parse() doesn’t accept single quotes.
So for example, this piece of code fails due to a trailing comma:
JSON.parse('{"age" : 10, }')
Output:
SyntaxError: Unexpected token } in JSON at position 13
As another example, parsing JSON with single quotes fails too:
JSON.parse("{'test': 1}")
Output:
SyntaxError: Unexpected token ' in JSON at position 1
Summary
Today you learned how to parse JSON in JavaScript.
To recap, use the built-in JSON.parse() function to parse JSON data by passing the JSON object as an argument to this function.